#include math.h
#include string
using namespace std;
class CPoint {
protected:
double m_x, m_y;
public:
CPoint(double x, double y) { m_x = x; m_y = y; }
virtual double Compute_Unit() = 0;
virtual void Print_Point()
{
cout << "(x : " << m_x << " y : " << m_y;
}
};
class CLengthPoint :public CPoint {
double m_z;
public:
CLengthPoint(double x, double y, double z) : CPoint(x, y) { m_z = z; }
double Compute_Unit();
void Print_Point()
{
CPoint::Print_Point();
cout << " z : " << m_z << ")\n";
}
};
double CLengthPoint::Compute_Unit()
{
return sqrt(m_x*m_x + m_y*m_y + m_z*m_z);
}
class CVoulmePoint :public CPoint {
protected:
double m_z;
public:
CVoulmePoint(double x, double y, double z) :CPoint(x, y) { m_z = z; }
double Compute_Unit();
void Print_Point()
{
CPoint::Print_Point();
cout <<" z : " << m_z << ")\n";
}
};
double CVoulmePoint::Compute_Unit()
{
if (m_x >= 0 && m_y >= 0 && m_z >= 0)
return m_x*m_y*m_z;
else
return 0;
}
class CAreaPoint :public CVoulmePoint {
public:
CAreaPoint(double x, double y, double z) :CVoulmePoint(x,y,z) {}
double Compute_Unit();
};
double CAreaPoint::Compute_Unit()
{
if (m_x >= 0 && m_y >= 0 && m_z >= 0)
return 2 * (m_x*m_y + m_y*m_z + m_z*m_x);
else
return 0;
}
int main()
{
cout << fixed;
cout.precision(1);
CPoint *pointer;
CLengthPoint lengthPt(5.7, 12.5, 3.4);
CVoulmePoint volumePt(11.6, 4.1, 5.4);
CAreaPoint areaPt(3.7, 8.9, 4.5);
double a = 0;
pointer = &lengthPt;
cout << "객체 lengthPt의 좌표 : ";
pointer->Print_Point();
a = pointer->Compute_Unit();
cout << "객체 lengthPt의 원점으로부터의 거리 : " << a << endl;
pointer = &volumePt;
cout << "객체 volumePt의 좌표 : ";
pointer->Print_Point();
a = pointer->Compute_Unit();
cout << "객체 volumePt의 육면체 부피 : " << a << endl;
pointer = &areaPt;
cout << "객체 areaPt의 좌표 : ";
pointer->Print_Point();
a = pointer->Compute_Unit();
cout << "객체 areaPt의 육면체 겉넓이 : " << a << endl;
return 0;
}
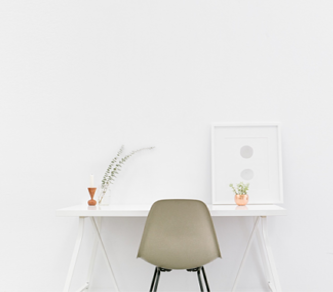