#include string
using namespace std;
class Employee {
protected:
string m_name;
int m_payRate;
float m_hoursWorked;
public:
Employee();
Employee(string name, int payRate, float hoursWorked);
void Set_Name(string name) { m_name = name; }
string Get_Name() { return m_name; }
void Set_PayRate(int payRate) { m_payRate = payRate; }
int Get_PayRate() { return m_payRate; }
void Set_HoursWorked(float hoursWorked) { m_hoursWorked = hoursWorked; }
float Get_HoursWorked() { return m_hoursWorked; }
float Compute_Salary();
void Print_Info();
};
Employee::Employee()
{
m_name = "NONE";
m_payRate = 0;
m_hoursWorked = 0.0;
}
Employee::Employee(string name, int payRate, float hoursWorked)
{
m_name = name;
m_payRate = payRate;
m_hoursWorked = hoursWorked;
}
float Employee::Compute_Salary()
{
return m_payRate*m_hoursWorked;
}
void Employee::Print_Info()
{
cout << "이름 : " << m_name << endl;
cout << "시간당 급여 : " << m_payRate << "원" << endl;
cout << "근무 시간 : " << m_hoursWorked << "시간" << endl;
cout << "총급여 : " << Compute_Salary() << "원" << endl << endl;
}
class Manager :public Employee
{
bool m_fullTime;
public:
Manager();
Manager(string name, int payRate, float hoursWorked, bool fullTime);
bool Get_FullTime() { return m_fullTime; }
void Set_FullTime(bool fullTime) { m_fullTime = fullTime; }
float Compute_Salary();
void Print_Info();
};
Manager::Manager()
{
m_fullTime = true;
}
Manager::Manager(string name, int payRate, float hoursWorked, bool fullTime):Employee(name, payRate, hoursWorked)
{
m_fullTime = fullTime;
}
float Manager::Compute_Salary()
{
if (m_fullTime == true)
{
Set_HoursWorked(40.0);
return Get_PayRate()*(1.5)*Get_HoursWorked();
}
else
return Employee::Compute_Salary();
}
void Manager::Print_Info()
{
cout << "이름 : " << Get_Name() << endl;
cout << "시간당 급여 : " << Get_PayRate() << "원" << endl;
if (m_fullTime == true)
{
cout << "졍규직 매니저" << endl;
cout << "근무시간 40시간 고정" << endl;
}
else
{
cout << "비졍규직 매니저" << endl;
cout << "근무 시간 : "<
cout << "총급여 : " << Compute_Salary() <<"원"<< endl << endl;
}
int main()
{
Employee ob1("장길산", 8000, 32.0);
Manager ob2("홍길동", 12000, 28.0, true);
cout << "<종업원>\n";
ob1.Print_Info();
ob1.Set_PayRate(8500);
ob1.Set_HoursWorked(40.0);
cout << "***** 종업원 PayPate 및 hours 변경 *****\n";
cout << "시간당 급여 : " << ob1.Get_PayRate() << "원" << endl;
cout << "근무 시간 : " << ob1.Get_HoursWorked() << "시간" << endl;
cout << "총급여 : " << ob1.Compute_Salary() << "원" << endl << endl;
cout << "<매니저1>\n";
ob2.Print_Info();
ob2.Set_PayRate(13500);
ob2.Set_HoursWorked(35.0);
cout << "***** 매니저 PayPate 및 hours 변경 *****\n";
cout << "시간당 급여 : " << ob2.Get_PayRate() << "원" << endl;
cout << "총급여 : " << ob2.Compute_Salary() << "원" << endl << endl;
cout << "<매니저2>\n";
Manager ob3;
ob3.Print_Info();
ob3.Set_Name("박문수");
ob3.Set_PayRate(12000);
ob3.Set_HoursWorked(25.5);
ob3.Set_FullTime(false);
cout << "***** 매니저 이름, PayPate 및 hours 변경 *****\n";
ob3.Print_Info();
return 0;
}
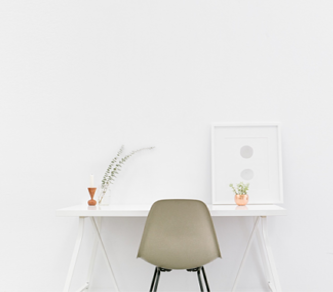