#include string
using namespace std;
class MyTime {
int m_hour;
int m_min;
public:
MyTime(int min = 0);
MyTime(int hour, int min);
MyTime operator+(MyTime t);
friend MyTime operator+(MyTime t, int min);
MyTime operator-(int min);
friend MyTime operator-(MyTime t1, MyTime t2);
MyTime operator*(double k);
friend MyTime operator*(double k, MyTime t);
MyTime operator=(MyTime t);
friend int operator>(MyTime t1, MyTime t2);
void Print_Time(string obj);
friend int Compute_Total_Mins(MyTime t);
MyTime Make_Time_Object(int min);
};
MyTime::MyTime(int min)
{
if (min < 0)
{
m_min = 0;
m_hour = 0;
}
else
{
m_hour = min / 60;
m_min = min % 60;
}
}
MyTime::MyTime(int hour, int min)
{
if (hour < 0 || min < 0)
{
m_hour = 0;
m_min = 0;
}
else
{
m_hour = hour + (min / 60);
if ((min > 60))
m_min = min % 60;
else
m_min = min;
}
}
MyTime MyTime::operator+(MyTime t)
{
MyTime temp; int a, b;
a=Compute_Total_Mins(*this);
b=Compute_Total_Mins(t);
return temp.Make_Time_Object(a + b);
}
MyTime operator+(MyTime t, int min)
{
MyTime temp; int a;
a = Compute_Total_Mins(t);
return temp.Make_Time_Object(a + min);
}
MyTime MyTime::operator-(int min)
{
MyTime temp; int a;
a = Compute_Total_Mins(*this);
if((a-min)>0)
return temp.Make_Time_Object(a - min);
else
{
temp.m_hour = 0;
temp.m_min = 0;
return temp;
}
}
MyTime operator-(MyTime t1, MyTime t2)
{
MyTime temp; int a = 0, b = 0, c = 0;
a=Compute_Total_Mins(t1);
b=Compute_Total_Mins(t2);
if (a > b)
return temp.Make_Time_Object(a - b);
else
{
temp.m_min = 0;
temp.m_hour = 0;
return temp;
}
}
MyTime MyTime::operator*(double k)
{
MyTime temp; int a;
a = Compute_Total_Mins(*this);
return temp.Make_Time_Object((int)(a * k));
}
MyTime operator*(double k, MyTime t)
{
MyTime temp; int a;
a = Compute_Total_Mins(t);
return temp.Make_Time_Object((int)(a * k));
}
MyTime MyTime::operator=(MyTime t)
{
m_hour = t.m_hour;
m_min = t.m_min;
return *this;
}
int operator>(MyTime t1, MyTime t2)
{
int a = 0, b = 0;
a = Compute_Total_Mins(t1);
b = Compute_Total_Mins(t2);
if (a > b)
return 1;
else if (a == b)
return 0;
else
return -1;
}
void MyTime::Print_Time(string obj)
{
if(m_hour>0 || m_min>0)
cout << obj << " : " << m_hour << "시간 " << m_min << "분\n";
else
cout << obj << " : " << m_min << "분\n";
}
int Compute_Total_Mins(MyTime t)
{
return (t.m_hour * 60) + t.m_min;
}
MyTime MyTime::Make_Time_Object(int min)
{
MyTime temp;
temp.m_hour = m_hour + (min / 60);
temp.m_min = m_min +(min % 60);
return temp;
}
int main()
{
MyTime ob1(135), ob2(2, 90), ob3(3, -30), result;
int a = 0, b = 0;
ob1.Print_Time("ob1");
ob2.Print_Time("ob2");
ob3.Print_Time("ob3");
a = Compute_Total_Mins(ob1);
b = Compute_Total_Mins(ob2);
cout << "ob1의 total mins : " << a << "분" << endl;
cout << "ob2의 total mins : " << b << "분" << endl;
result = ob1 + ob2;
result.Print_Time("ob1 + ob2");
result = ob1 + 80;
result.Print_Time("ob1 + 80");
result = ob1 - ob2;
result.Print_Time("ob1 - ob2");
result = ob2 - ob1;
result.Print_Time("ob2 - ob1");
result = ob1 - 45;
result.Print_Time("ob1 - 45");
result = ob2 * 10;
result.Print_Time("ob1 * ob2");
result = 3.75 * ob2;
result.Print_Time("3.75 * ob2");
switch (ob1>ob2)
{
case 1:cout << "ob1의 시간은 ob2보다 큽니다\n"; break;
case 0:cout << "ob1의 시간은 ob2와 같습니다\n"; break;
case -1:cout << "ob1의 시간은 ob2보다 작습니다\n"; break;
}
result = ob1 = ob2;
result.Print_Time("ob1 = ob2");
switch (ob1>ob2)
{
case 1:cout << "ob1의 시간은 ob2보다 큽니다\n"; break;
case 0:cout << "ob1의 시간은 ob2와 같습니다\n"; break;
case -1:cout << "ob1의 시간은 ob2보다 작습니다\n"; break;
}
return 0;
}
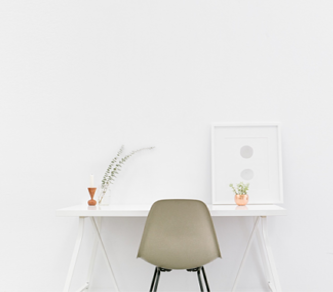