#include string
using namespace std;
void Input_Data(int *money, int *type, int *num);
int Compute_total(int type, int num);
char Check_Money(int total, int *money);
void Print_Result(char selection, int total, int money, int type, int num);
int main(void)
{
int money = 0, type = 0, num = 0, total=0;
char selection = 0;
do
{
Input_Data(&money, &type, &num);
total = Compute_total(type, num);
selection = Check_Money(total, &money);
Print_Result(selection, total, money, type, num);
cout << "\n음료수를 더 뽑겠습니까? (Y/N):";
cin >> selection;
while (selection != 'y' && selection != 'Y'&& selection != 'n' && selection != 'N')
{
cout << "오직 Y,y,N,n만 입력하시오 : ";
cin >> selection;
}
}while (selection == 'y' || selection == 'Y');
cout << "이용해 주셔서 감사합니다." << endl;
return 0;
}
void Input_Data(int *money, int *type, int *num)
{
cout << "=======음료 자판기===========" << endl;
cout << "1. 생수 : 500원 2.사이다 : 800원 3.옥수수차 : 1200원" << endl;
cout << "===========================" << endl;
cout << "돈을 넣어 주세요: ";
cin >> *money;
cout << "음료 선택 및 수량: ";
cin >> *type >> *num;
}
int Compute_total(int type, int num)
{
int total = 0;
switch (type)
{
case 1: total = 500 * num; break;
case 2: total = 800 * num; break;
case 3: total = 1200 * num; break;
}
return total;
}
char Check_Money(int total, int *money)
{
int a = 0;
char selection = 0;
while (total>*money)
{
cout << "금액이 " << total - *money << "원 부족합니다.돈을 더 투입하시겠습니까?(Y/N)";
cin >> selection;
while (selection != 'y' && selection != 'Y'&& selection != 'n' && selection != 'N')
{
cout << "오직 Y,y,N,n만 입력하시오 : ";
cin >> selection;
}
if (selection == 'n' || selection == 'N')
{
cout << "투입하신 금액이 부족하여 판매가 취소되었습니다." << endl;
break;
}
cout << "추가 투입금:";
cin >> a;
*money += a;
}
return selection;
}
void Print_Result(char selection, int total, int money, int type, int num)
{
string arr[3] = { "생수","사이다","옥수수차" };
if (selection == 'n' || selection == 'N')
cout << "투입하신 돈 " << money << "원을 받으십시요" << endl;
else
{
cout << "현재 금액: " << money << "입니다." << endl;
cout << arr[type-1] << num << "개와 잔돈 " << money - total << "을 받으십시오" << endl;
}
}
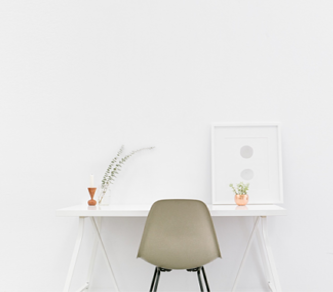