Student
package org.javaro.lecture;
public class Student {
private String name;
public Student() {}
public String getName() {
return this.name;
}
public void setName(String name) {
this.name=name;
}
public String toString() {
return "학생이름="+this.getName();
}
}
Book
package org.javaro.lecture;
public class Book {
private String title; private String author; private Student student;
public Book(String title) {this.title=title;}
public void setTitle(String title) {this.title=title;}
public String getTitle() {return this.title;}
public void setAuthor(String author) {this.author=author;}
public String getAutor() {return this.author;}
public void setStudent(Student student) {this.student=student;}
public Student getStudent() {return this.student;}
public String toString() {
String available;
if(this.getStudent()==null) {
available="대출가능";
}else {
available="대출자="+this.getStudent().getName();
}
return"제목="+this.getTitle()+",저자="+this.getAutor()+","+available;
}
}
BookStroe
package org.javaro.lecture;
import java.util.ArrayList;
public class BookStroe {
public String storeName;
public ArrayListbooks;
public ArrayList students;
public BookStroe(String storeName) {
this.storeName = storeName;
books = new ArrayList();
students = new ArrayList();
}
public String getStoreName() {
return this.storeName;
}
public ArrayListgetBooks(){
return this.books;
}
public ArrayList getStudent(){
return this.students ;
}
public void addBook(Book book) {
this.books.add(book);
}
public void removeBook(Book book) {
this.books.remove(book);
}
public void addStudent(Student student) {
this.students.add(student);
}
public void removeStudent(Student student) {
this.students.remove(student);
}
public boolean checkOut(Book book, Student student) {
if(book.getStudent()==null) {
book.setStudent(student);
return true;
}else {
return false;
}
}
public boolean checkln(Book book) {
if(book.getStudent()!=null) {
book.setStudent(null);
return true;
}else {
return false;
}
}
public ArrayList getBooksForStudent(Student student){
ArrayListresult = new ArrayList();
for(Book aBook : this.getBooks()) {
if((aBook.getStudent()!=null)&&(aBook.getStudent().equals(student.getName()))) {
result.add(aBook);
}
}
return result;
}
public ArrayList getAvaliableBooks(){
ArrayListresult=new ArrayList();
for(Book aBook : this.getBooks()) {
if(aBook.getStudent()==null) {
result.add(aBook);
}
}
return result;
}
public ArrayList getUnavailableBooks(){
ArrayList result=new ArrayList();
for(Book aBook : this.getBooks()) {
if(aBook.getStudent()!=null) {
result.add(aBook);
}
}
return result;
}
public String toString() {
return this.getStoreName()+"의 보유책="+this.getBooks().size()+"권, 회원수="+ this.getStudent().size()+"명";
}
public void printStatus() {
System.out.println("--도서관 현황--\n"+this.toString());
for(Book aBook : this.getBooks()) {
System.out.println(aBook);
}
for(Student student : this.getStudent()) {
int count = this.getBooksForStudent(student).size();
System.out.println(student+"은/는"+count+"권 대출중");
}
System.out.println("현재 대출 가능 책: "+this.getAvaliableBooks().size());
System.out.println("--리포트 종료--");
}
}
Mystore
package org.javaro.lecture;
import java.util.ArrayList;
import org.javaro.lecture.*;
public class Mystore {
public static void main (String[] args) {
System.out.println("조병수-20191127-응용");
BookStroe myStore = new BookStroe("도서관");
Book book1 = new Book("홍길동전"); Book book2 = new Book("심청전");
book1.setAuthor("허균"); book2.setAuthor("미상");
Student stud1 = new Student();
Student stud2 = new Student();
stud1.setName("이몽룡"); stud2.setName("변학도");
myStore.addBook(book1); myStore.addBook(book2);
myStore.addStudent(stud1); myStore.addStudent(stud2);
System.out.println("도서 관리 시스템 생성");
myStore.printStatus();
System.out.println("book1 홍길동전을 stud2 변학도에게 대출");
myStore.checkOut(book1,stud2);
myStore.printStatus();
System.out.println("book1 홍길동전 반납");
myStore.checkln(book1);
System.out.println("book2 심청전을 stud1 이몽룡에게 대출");
myStore.checkOut(book2,stud1);
myStore.printStatus();
}
}
실행창
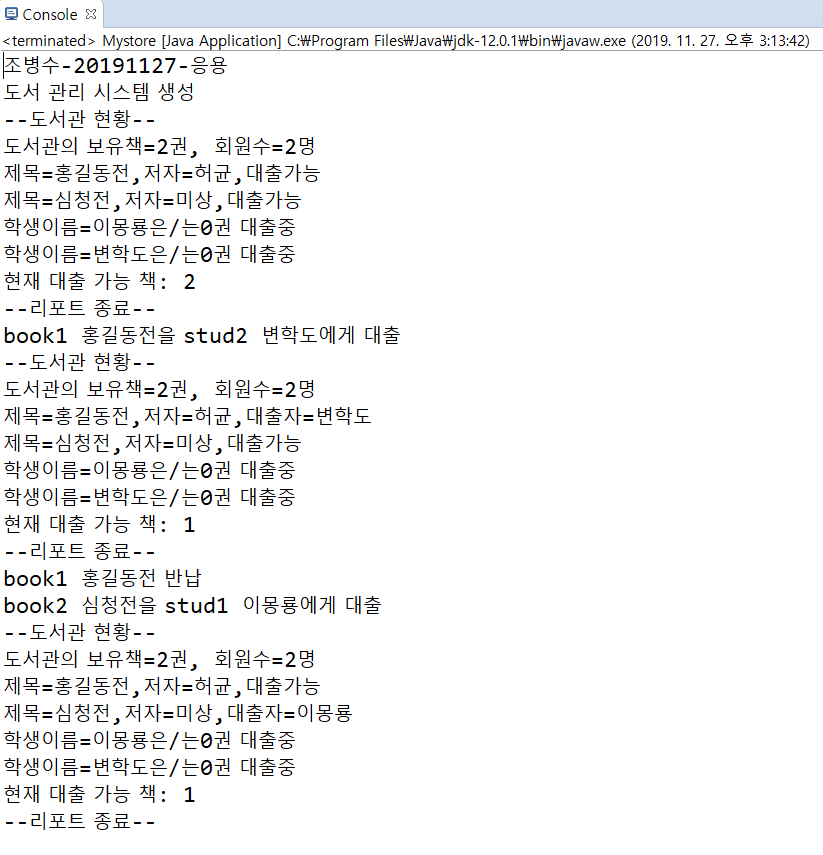